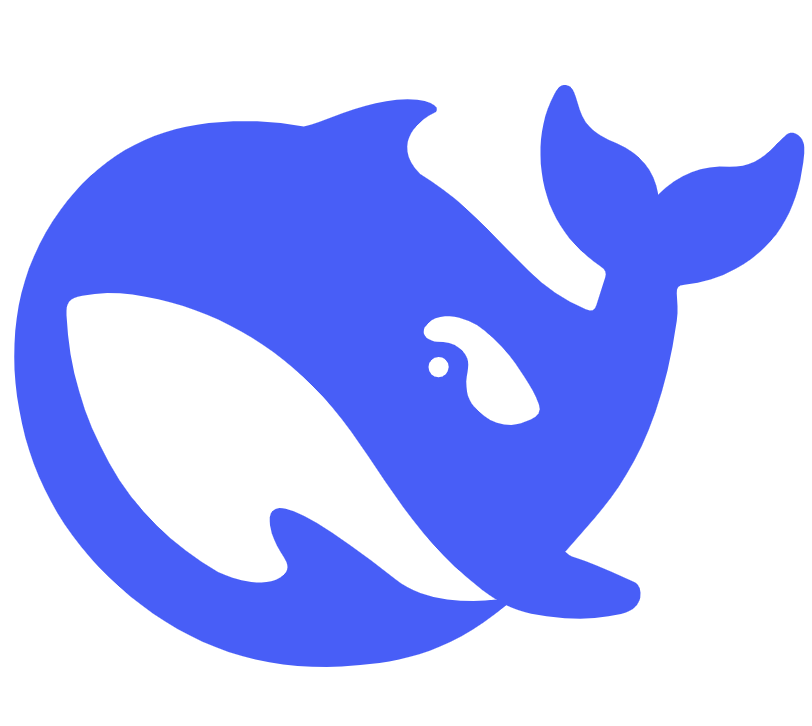
There are three main ways that you can iterate through a java map
- Use entrySet() function
public void iterateByEntrySet(Map map) {
for (Map.Entry entry : map.entrySet()) {
System.out.println(entry.getKey() + ":" + entry.getValue());
}
}
2. Use ketSet() function
public void iterateByKeySetAndForeach(Map map) {
for (String key : map.keySet()) {
System.out.println(key + ":" + map.get(key));
}
}
3. Use Values() function (Iterate over the values regardless of the key)
public void iterateValues(Map map) {
for (Integer value : map.values()) {
System.out.println(value);
}
}
The followings are some more example operations on java map using java stream API
eg 1: filter map using map value field and build comma separated string.
eg 2: filter map using map key and collect the result to a new map
Map map = new HashMap<>();
map.put(1, "a");
map.put(2, "b");
//filter map using map value field and build comma seperated string.
String result = map.entrySet().stream()
.filter(x -> "something".equals(x.getValue()))
.map(x->x.getValue())
.collect(Collectors.joining());
//filter map using map key and collect the result to a new map
Map collect = map.entrySet().stream()
.filter(x -> x.getKey() == 2)
.collect(Collectors.toMap(x -> x.getKey(), x -> x.getValue()));
Map collect = map.entrySet().stream()
.filter(x -> x.getKey() == 3)
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
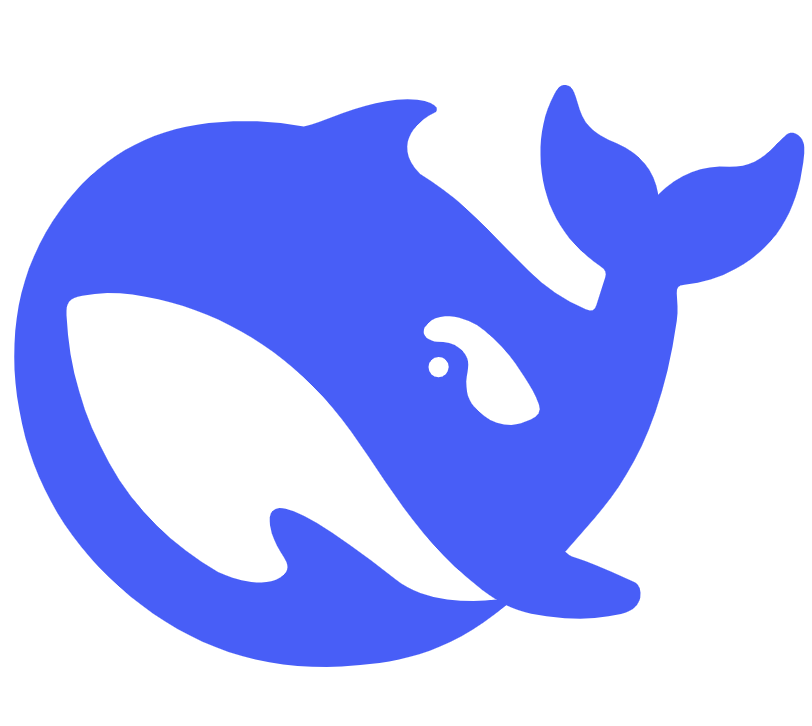
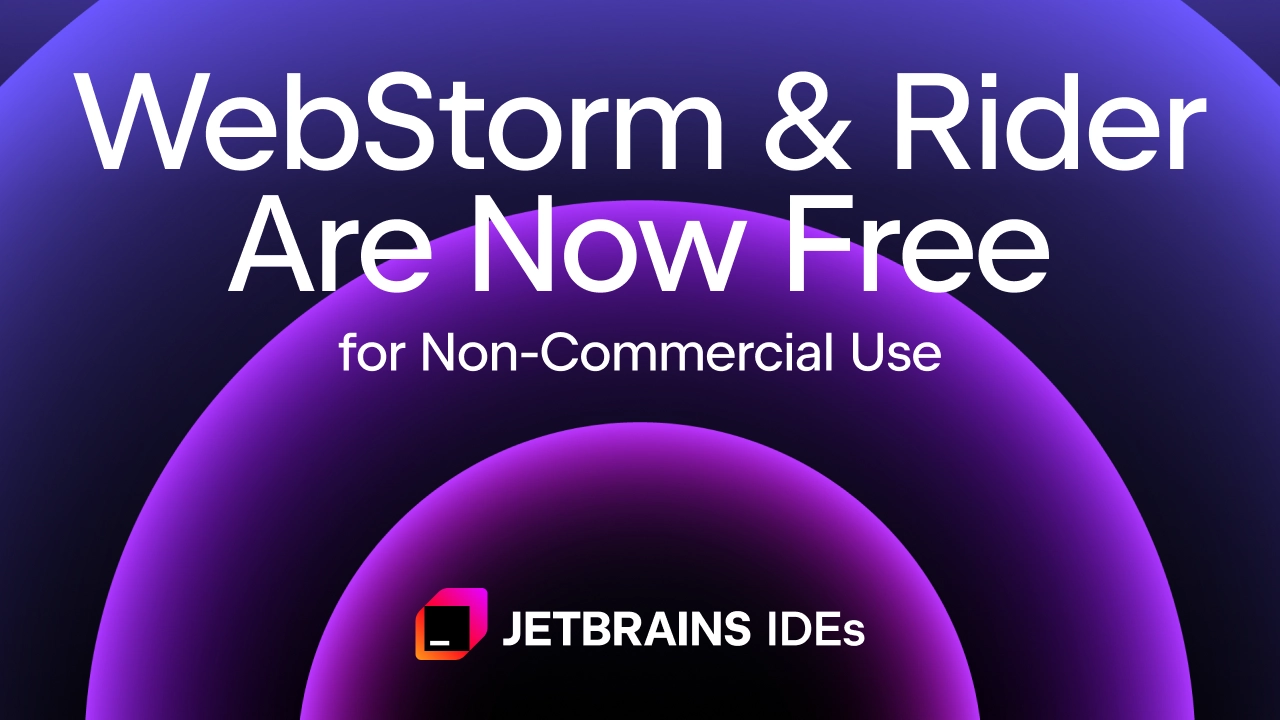
JetBrains Announces Free Non-Commercial Licensing for WebStorm and Rider
October 25, 2024
No Comments
Read More »
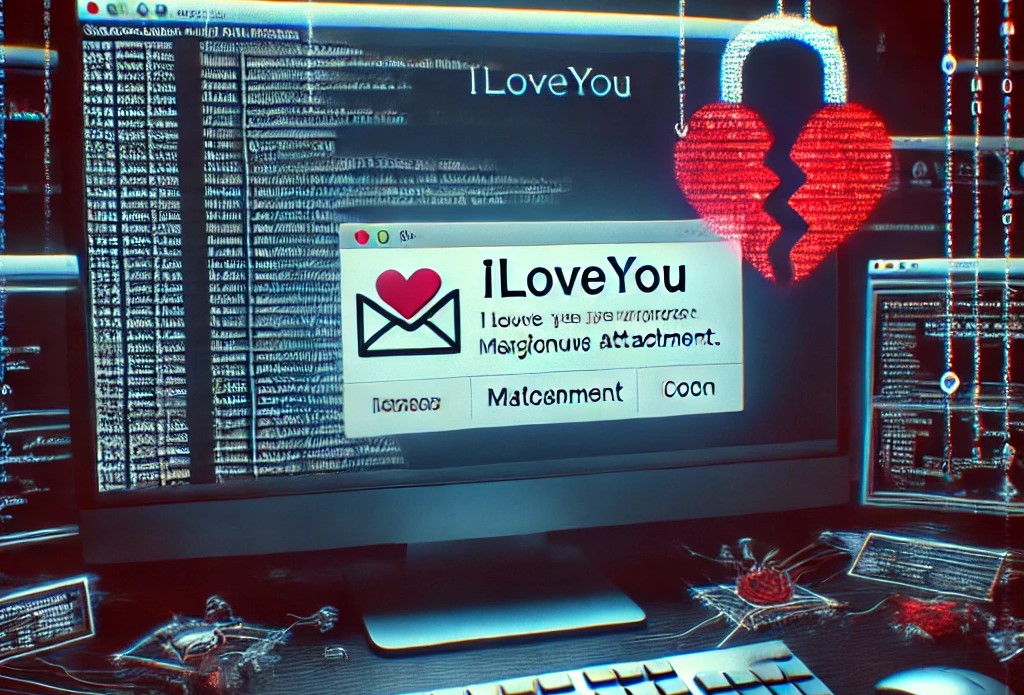
The LoveLetter Virus: The Infamous Cyberattack That Shook the World
October 8, 2024
No Comments
Read More »
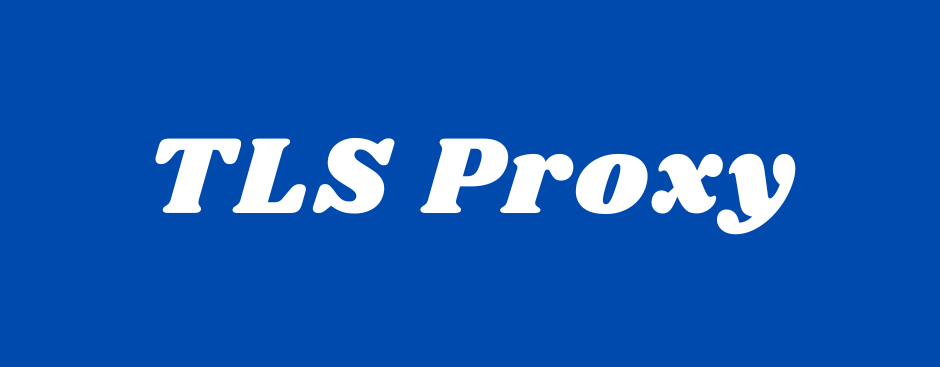
Understanding TLS Proxies: How They Work and Why They’re Important
August 11, 2024
No Comments
Read More »
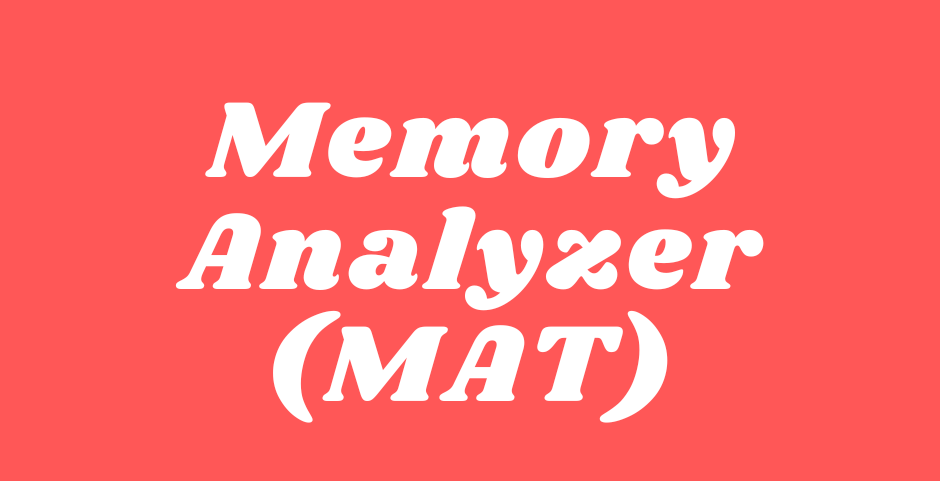
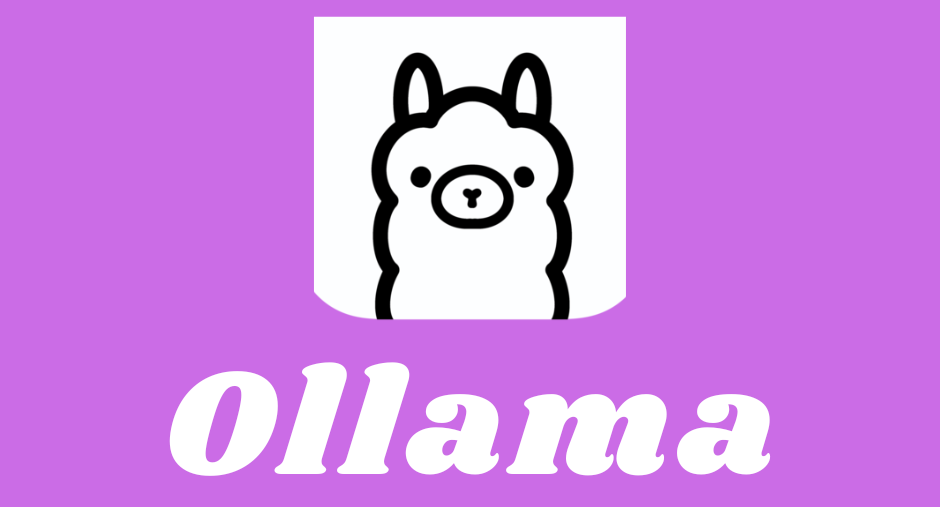
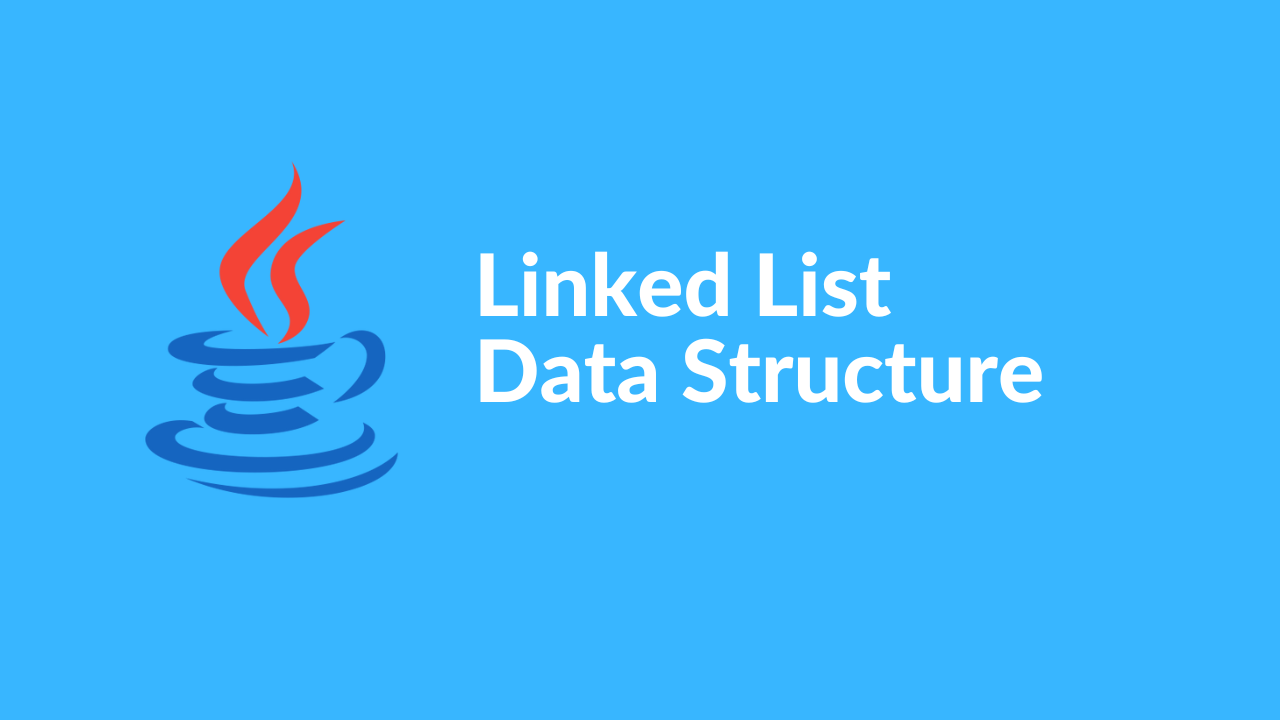
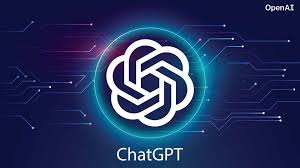
How to iterate any Map in Java