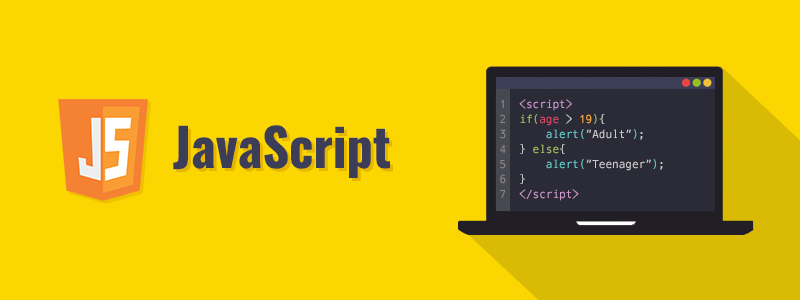
Serialization and deserialization are two important concepts in Java that allow objects to be converted into a stream of bytes and then retrieved back into objects again. In this article, we will discuss what serialization and deserialization are, why they are important, and how to implement them in Java.
What is Serialization?
Serialization is the process of converting an object into a stream of bytes, which can then be stored in a file or sent over a network. The serialized object includes the object’s data and class information, which can be used to recreate the object at a later time.
In Java, serialization is implemented using the Serializable interface, which is a marker interface that indicates that a class can be serialized. When an object of a serializable class is serialized, its data is written to a stream of bytes that can be saved to a file or sent over a network.
What is Deserialization?
Deserialization is the reverse process of serialization. It involves converting a stream of bytes back into an object, which can then be used in a Java program. The deserialized object is an exact copy of the serialized object, including all of its data and class information.
In Java, deserialization is implemented using the readObject() method, which is a method of the ObjectInputStream class. This method reads the serialized data from a stream of bytes and then recreates the object.
Why is Serialization and Deserialization Important?
Serialization and deserialization are important because they allow objects to be transferred between different systems or applications. For example, if you have an object in one Java program that you want to use in another program, you can serialize the object and then send it over the network to the other program. The other program can then deserialize the object and use it.
Serialization and deserialization are also important for saving objects to a file. By serializing an object, you can save its data to a file and then retrieve it later by deserializing the data.
Implementing Serialization and Deserialization in Java
To implement serialization and deserialization in Java, you need to follow these steps:
Implement the Serializable interface: To make a class serializable, it must implement the Serializable interface. This interface doesn’t have any methods that you need to implement; it just indicates that the class can be serialized.
Use ObjectOutputStream to serialize objects: To serialize an object, you need to use the ObjectOutputStream class. This class has a writeObject() method that writes the object to a stream of bytes.
Use ObjectInputStream to deserialize objects: To deserialize an object, you need to use the ObjectInputStream class. This class has a readObject() method that reads the object from a stream of bytes.
Here’s an example of serializing and deserializing an object in Java:
import java.io.*;
public class SerializationDemo {
public static void main(String[] args) {
// Serialize an object
try {
Employee emp = new Employee("John Doe", "Developer", 10000);
FileOutputStream fileOut = new FileOutputStream("employee.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(emp);
out.close();
fileOut.close();
System.out.println("Serialized data is saved in employee.ser");
} catch (IOException i) {
i.printStackTrace();
}
// Deserialize an object
try {
FileInputStream fileIn = new FileInputStream("employee.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Employee emp = (Employee) in.readObject();
in.close();
fileIn.close();
System.out.println("Deserialized data: ");
System.out.println(emp.getName());
System.out.println(emp.getRole());
System.out.println(emp.getSalary());
} catch (IOException i) {
i.printStackTrace();
return;
} catch (ClassNotFoundException c)
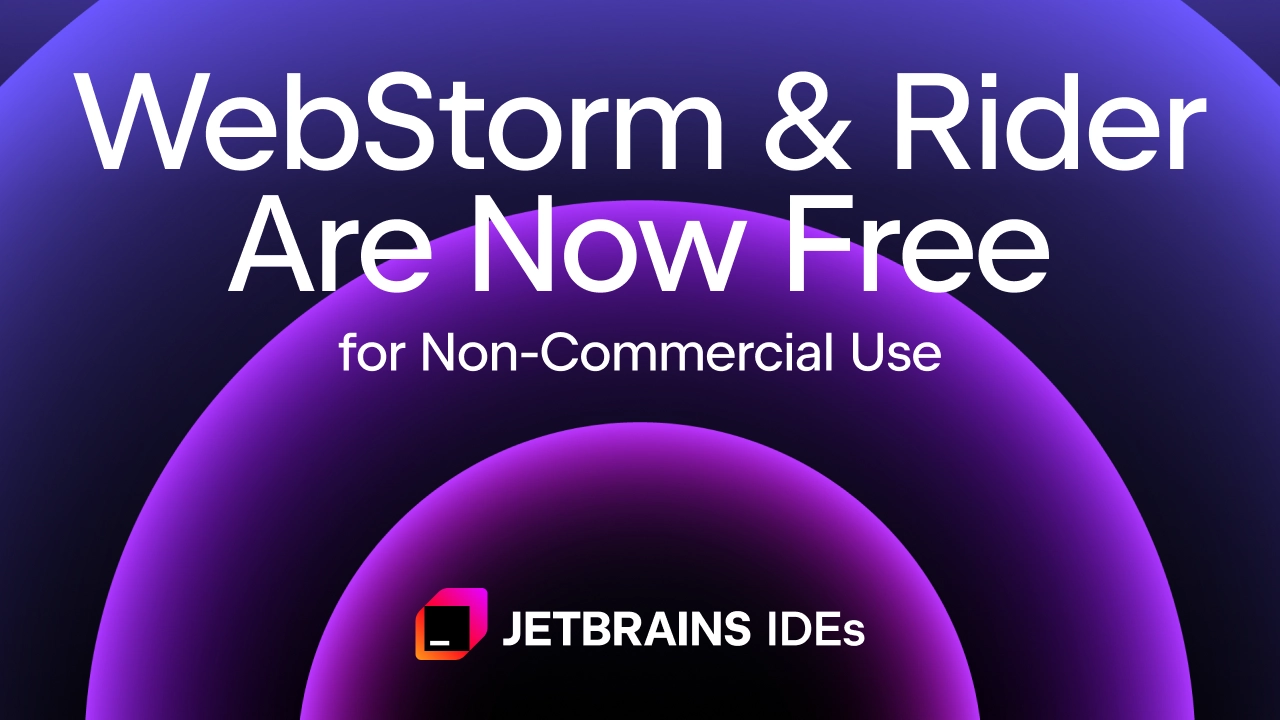
JetBrains Announces Free Non-Commercial Licensing for WebStorm and Rider
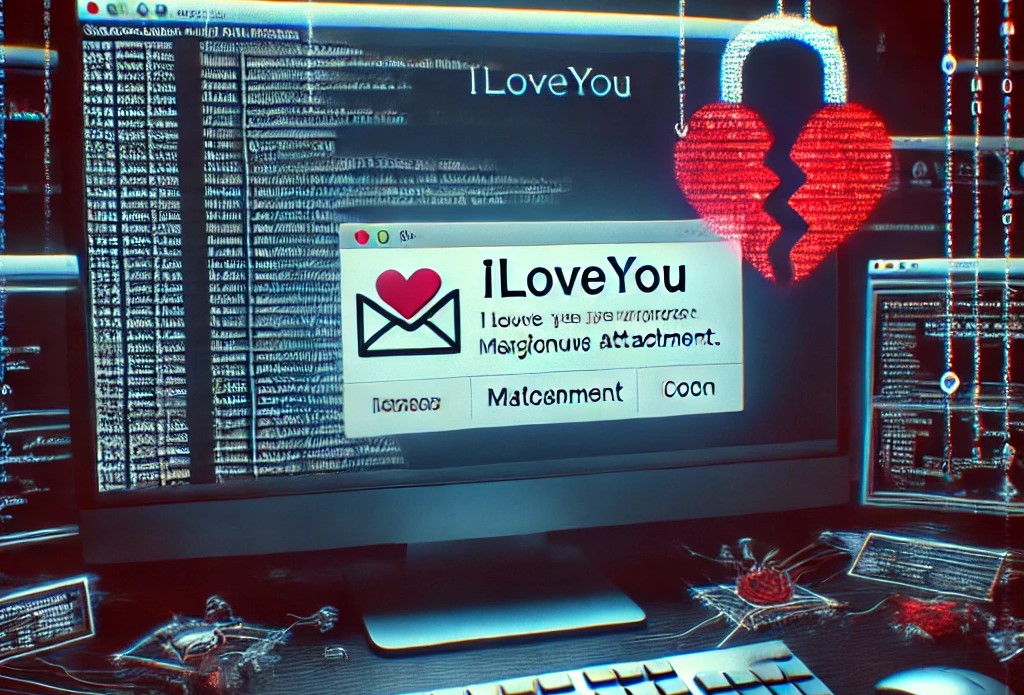
The LoveLetter Virus: The Infamous Cyberattack That Shook the World
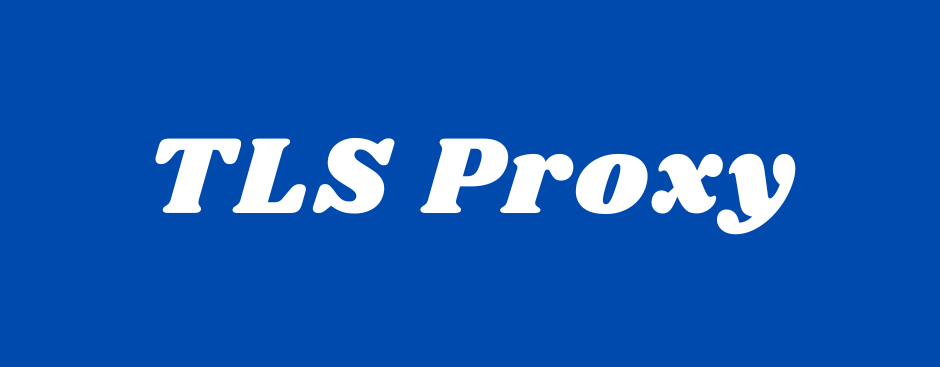
Understanding TLS Proxies: How They Work and Why They’re Important
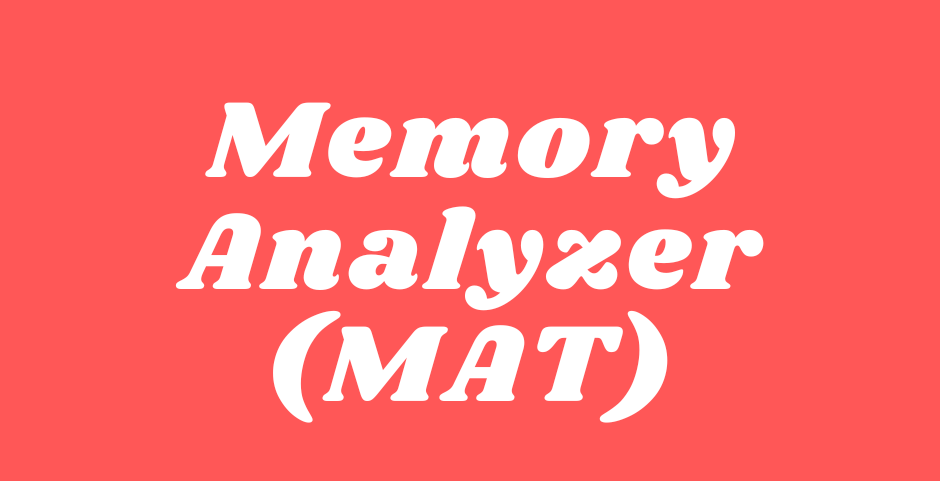
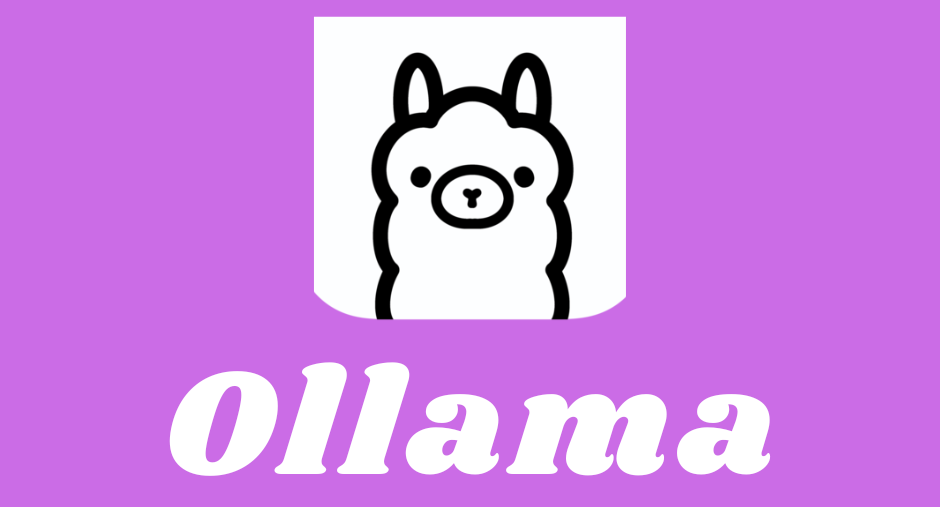
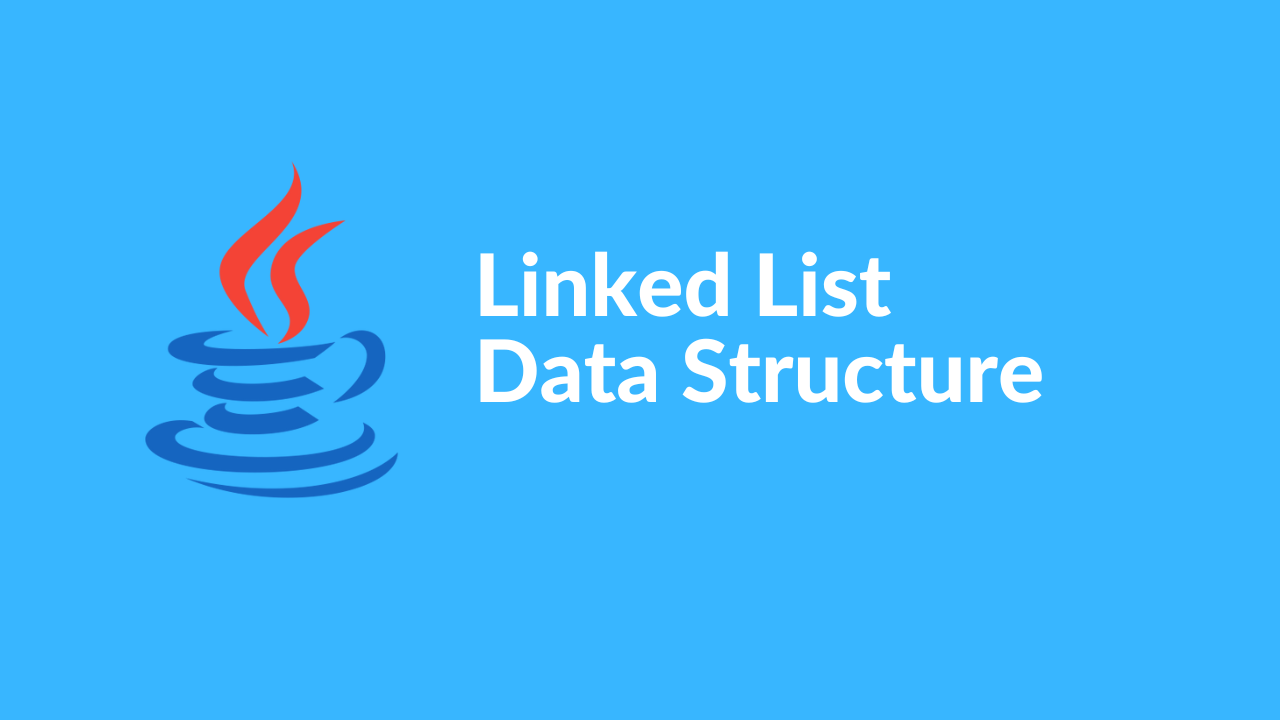
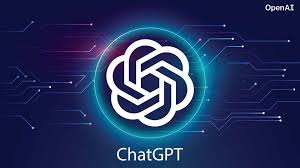
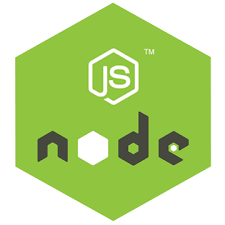