Writing Clean Code in a Java Spring Boot Application: Best Practices, Dos, and Don’ts
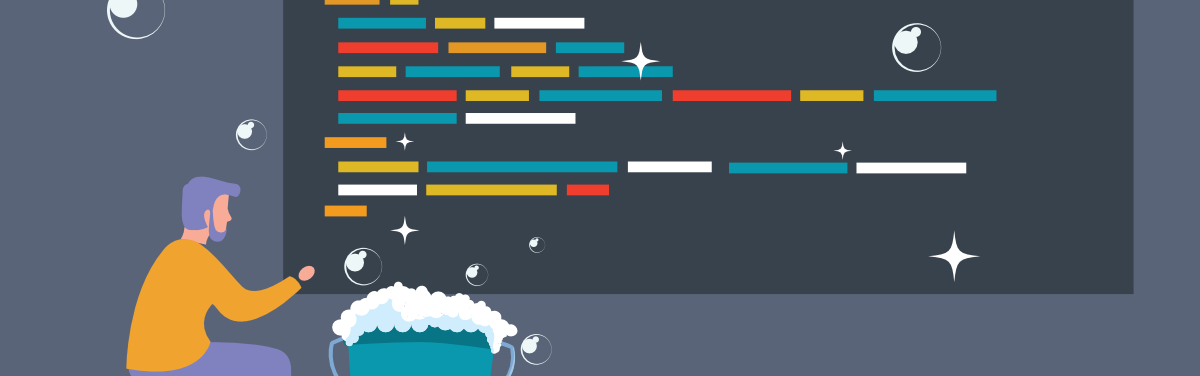
When building enterprise-grade applications, writing clean and maintainable code is crucial for long-term success. Java, coupled with Spring Boot, provides a robust framework for developing scalable applications. However, even the best tools need to be used effectively, which is where clean code practices come in.
In this blog post, we will explore the best practices for writing clean code in a Spring Boot application, along with the dos and don’ts to help you build high-quality software that is easier to maintain, extend, and debug.
Why Clean Code Matters
Clean code is:
- Easier to read: Both for you and your team. As your application grows, clarity becomes essential.
- Easier to maintain: Clean code reduces technical debt and helps prevent bugs from creeping into the system.
- Scalable: Clean code practices make it easier to add new features, refactor existing ones, and adapt to evolving requirements.
Best Practices for Clean Code in Spring Boot Applications
1. Follow the Single Responsibility Principle (SRP)
Each class, method, and module in your application should have only one responsibility. This aligns with the SOLID principles and promotes modular design.
Dos:
- Keep classes and methods small and focused.
- Ensure each service class in your Spring Boot application is handling only one type of functionality.
Don’ts:
- Don’t create “God” classes that handle multiple responsibilities. This leads to poor modularization and difficulty in understanding code.
Example:
// Good: A service focused on user management
@Service
public class UserService {
public void registerUser(UserDto user) { ... }
public User getUser(Long id) { ... }
}
2. Use Dependency Injection Wisely
Spring Boot’s primary strength lies in its dependency injection (DI) mechanism. This enhances testability and maintains loose coupling between components.
Dos:
- Use constructor-based dependency injection. It’s clearer and easier to test.
- Use
@Autowired
annotations sparingly, ideally only in constructors.
Don’ts:
- Avoid field injection. It makes unit testing difficult and introduces immutability issues.
Example:
// Good: Constructor-based dependency injection
@Service
public class OrderService {
private final OrderRepository orderRepository;
@Autowired
public OrderService(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
}
3. Adopt Consistent Naming Conventions
Use meaningful names that clearly describe the function of the variable, method, or class. Naming conventions improve readability and help others (or yourself, later on) quickly understand what each part of the code does.
Dos:
- Follow Java naming conventions (e.g., camelCase for variables, PascalCase for classes).
- Use domain-specific terms when naming classes and methods.
Don’ts:
- Don’t use abbreviations or single-character variable names that don’t convey meaning.
Example:
// Good: Clear and descriptive method names
public void createUserAccount(UserDto user) { ... }
4. Handle Exceptions Properly
Improper exception handling can lead to hidden bugs or inconsistent system behavior. Your Spring Boot application should handle exceptions gracefully, ensuring users don’t see raw stack traces.
Dos:
- Use custom exceptions to make your errors domain-specific.
- Implement global exception handling using
@ControllerAdvice
to avoid duplication. - Log meaningful error messages.
Don’ts:
- Don’t swallow exceptions without handling them, i.e., empty catch blocks.
- Avoid using generic exceptions like
Exception
orThrowable
in your catch blocks.
Example:
// Good: Custom exception handling
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity handleNotFound(ResourceNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
}
5. Write Readable and Maintainable Tests
Testing is crucial to ensure that your Spring Boot application behaves as expected. Write unit and integration tests that are clear and maintainable.
Dos:
- Follow a clear naming convention for your test methods.
- Test one thing at a time (avoid testing multiple functionalities in a single test case).
- Mock external dependencies (e.g., using Mockito or Spring’s
@MockBean
).
Don’ts:
- Don’t create overly complex tests. Your tests should be as simple and readable as possible.
Example:
// Good: Simple and descriptive test method
@Test
public void shouldReturnUserWhenExists() {
User user = new User("John", "john@example.com");
when(userRepository.findById(1L)).thenReturn(Optional.of(user));
User foundUser = userService.getUser(1L);
assertEquals("John", foundUser.getName());
}
6. Use Proper Annotations and Configuration
Spring Boot comes with a rich set of annotations that simplify configuration, but improper use can lead to performance issues or bugs.
Dos:
- Use
@Configuration
and@ComponentScan
to organize beans and configurations logically. - Use
@Transactional
only when necessary and at the appropriate granularity.
Don’ts:
- Don’t overuse
@Component
annotation, as it may introduce ambiguous bean definitions.
Example:
// Good: Transactional annotation at service level
@Service
public class PaymentService {
@Transactional
public void processPayment(PaymentRequest paymentRequest) {
// logic to process payment
}
}
7. Keep Your Code DRY (Don’t Repeat Yourself)
Duplicate code leads to higher maintenance costs and increases the chances of bugs. Use reusable components and abstraction where appropriate.
Dos:
- Extract common logic into reusable methods or classes.
- Use Spring Boot’s capabilities like
@ControllerAdvice
,@RestController
, or@Service
for reuse and consistency.
Don’ts:
- Avoid copy-pasting logic across different parts of the codebase.
Example:
// Good: Reusable method to handle validation
public boolean isValidEmail(String email) {
return email.matches("^[A-Za-z0-9+_.-]+@(.+)$");
}
8. Follow Layered Architecture
Stick to a clear separation of concerns within your application’s architecture, such as controllers, services, repositories, and models.
Dos:
- Use the layered architecture style: Controllers handle web requests, services contain business logic, and repositories manage data access.
- Keep each layer distinct and avoid cross-layer pollution.
Don’ts:
- Don’t put business logic in controllers or repositories.
Example:
// Good: Clear separation of layers
@Controller
public class ProductController {
private final ProductService productService;
public ProductController(ProductService productService) {
this.productService = productService;
}
@GetMapping("/products/{id}")
public Product getProduct(@PathVariable Long id) {
return productService.getProductById(id);
}
}
Conclusion
Writing clean code in a Spring Boot application requires discipline and adherence to best practices. By following the dos and don’ts listed above, you’ll build an application that’s easy to read, maintain, and scale. Clean code ultimately leads to fewer bugs, better team collaboration, and a more sustainable development process.
Remember, clean code is not just about following rules; it’s about writing code that you (and your teammates) will thank yourself for later!