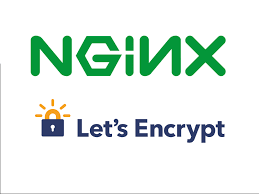
In this blog post, I’ll guide you through the process of converting an Excel sheet to a JSON file using Node.js. This can be incredibly useful for tasks such as data migration, data processing, or simply converting your data to a more accessible format.
Prerequisites
Before we begin, make sure you have Node.js installed on your computer. If not, you can download and install it from Node.js official website. You will also need to install the xlsx
package for reading Excel files and the fs
(file system) module for writing files.
Step 1: Setting Up Your Project
First, create a new directory for your project and navigate into it via your terminal or command prompt.
mkdir excel-to-json
cd excel-to-json
Next, initialize a new Node.js project:
npm init -y
This command will create a package.json
file with default settings.
Step 2: Install Required Packages
Install the xlsx
package, which allows us to read Excel files, and the fs
module, which is included in Node.js by default.
npm install xlsx
Step 3: Writing the Script
Create a new file named convert.js
in your project directory and open it in your preferred code editor. Add the following code to this file:
const xlsx = require('xlsx');
const fs = require('fs');
// Load the Excel file
const workbook = xlsx.readFile('path/to/your/excel.xlsx');
// Select the first sheet
const sheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[sheetName];
// Convert sheet to JSON
const jsonData = xlsx.utils.sheet_to_json(worksheet);
// Save JSON to a file
fs.writeFileSync('path/to/your/jsonfile.json', JSON.stringify(jsonData, null, 2));
console.log('JSON file saved');
Step 4: Running the Script
To run the script, use the following command in your terminal or command prompt:
node convert.js
If everything is set up correctly, you should see the message: JSON file saved
Conclusion
You’ve now successfully converted an Excel sheet to a JSON file using Node.js. This script can be modified to handle different Excel files and sheets as needed. The xlsx
package is powerful and supports various Excel operations, making it a valuable tool for your Node.js projects.
Feel free to experiment with the code and customize it to suit your specific requirements. Happy coding!