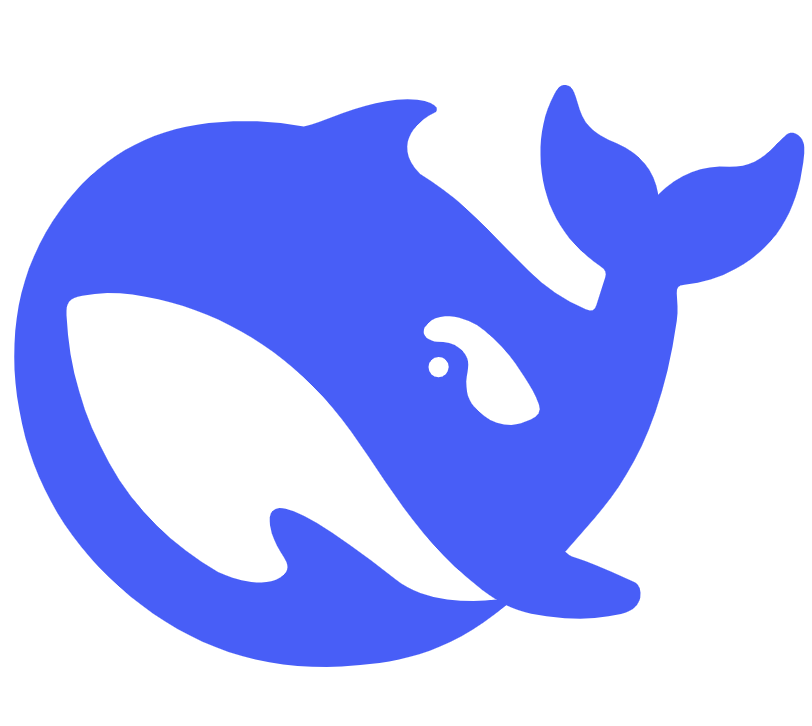
In this blog post, I’ll guide you through the process of integrating with the ChatGPT API to enable automated interactions with OpenAI’s powerful language model. Whether you’re looking to enhance your application with conversational capabilities or automate text generation tasks, the ChatGPT API provides a straightforward way to leverage the power of AI.
Prerequisites
Before getting started, ensure you have:
- A registered OpenAI account and an API key. You can sign up and get your API key from OpenAI.
- Node.js installed on your computer. If not, download and install it from Node.js official website.
Step 1: Setting Up Your Project
First, create a new directory for your project and navigate into it via your terminal or command prompt.
mkdir chatgpt-integration
cd chatgpt-integration
Next, initialize a new Node.js project:
npm init -y
This command will create a package.json
file with default settings.
Step 2: Install Required Packages
Install the axios
package for making HTTP requests and dotenv
for managing environment variables.
npm install axios dotenv
Step 3: Create a .env File
Create a .env
file in your project directory to securely store your OpenAI API key.
OPENAI_API_KEY=your_openai_api_key_here
Replace your_openai_api_key_here
with your actual OpenAI API key.
Step 4: Writing the Script
Create a new file named chatgpt.js
in your project directory and open it in your preferred code editor. Add the following code to this file:
const axios = require('axios');
require('dotenv').config();
const apiKey = process.env.OPENAI_API_KEY;
const apiUrl = 'https://api.openai.com/v1/chat/completions';
async function getChatGPTResponse(prompt) {
try {
const response = await axios.post(
apiUrl,
{
model: 'gpt-4',
messages: [{ role: 'user', content: prompt }],
max_tokens: 150,
},
{
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json',
},
}
);
const message = response.data.choices[0].message.content;
console.log('ChatGPT Response:', message);
return message;
} catch (error) {
console.error('Error fetching response:', error.response ? error.response.data : error.message);
}
}
// Example prompt
const prompt = 'Tell me a joke about programming.';
getChatGPTResponse(prompt);
Step 5: Running the Script
To run the script, use the following command in your terminal or command prompt:
node chatgpt.js
Example Prompt
const prompt = 'Tell me a joke about programming.';
getChatGPTResponse(prompt);
Conclusion
You’ve now successfully integrated with the ChatGPT API using Node.js. This script can be modified to handle different prompts and integrate with various applications. The ChatGPT API is a powerful tool that can enhance your projects with conversational AI capabilities. Feel free to experiment with the code and customize it to suit your specific needs. Happy coding!
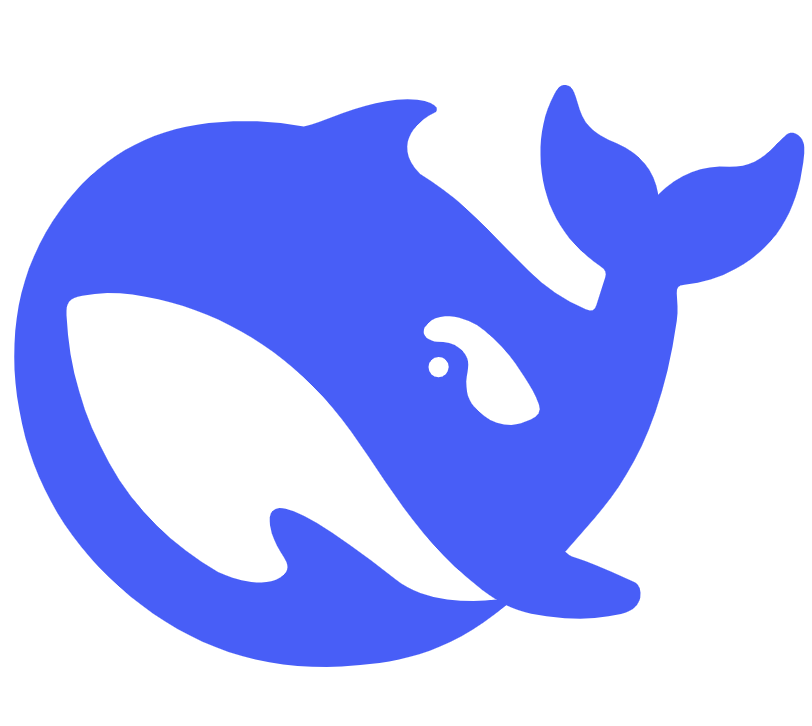
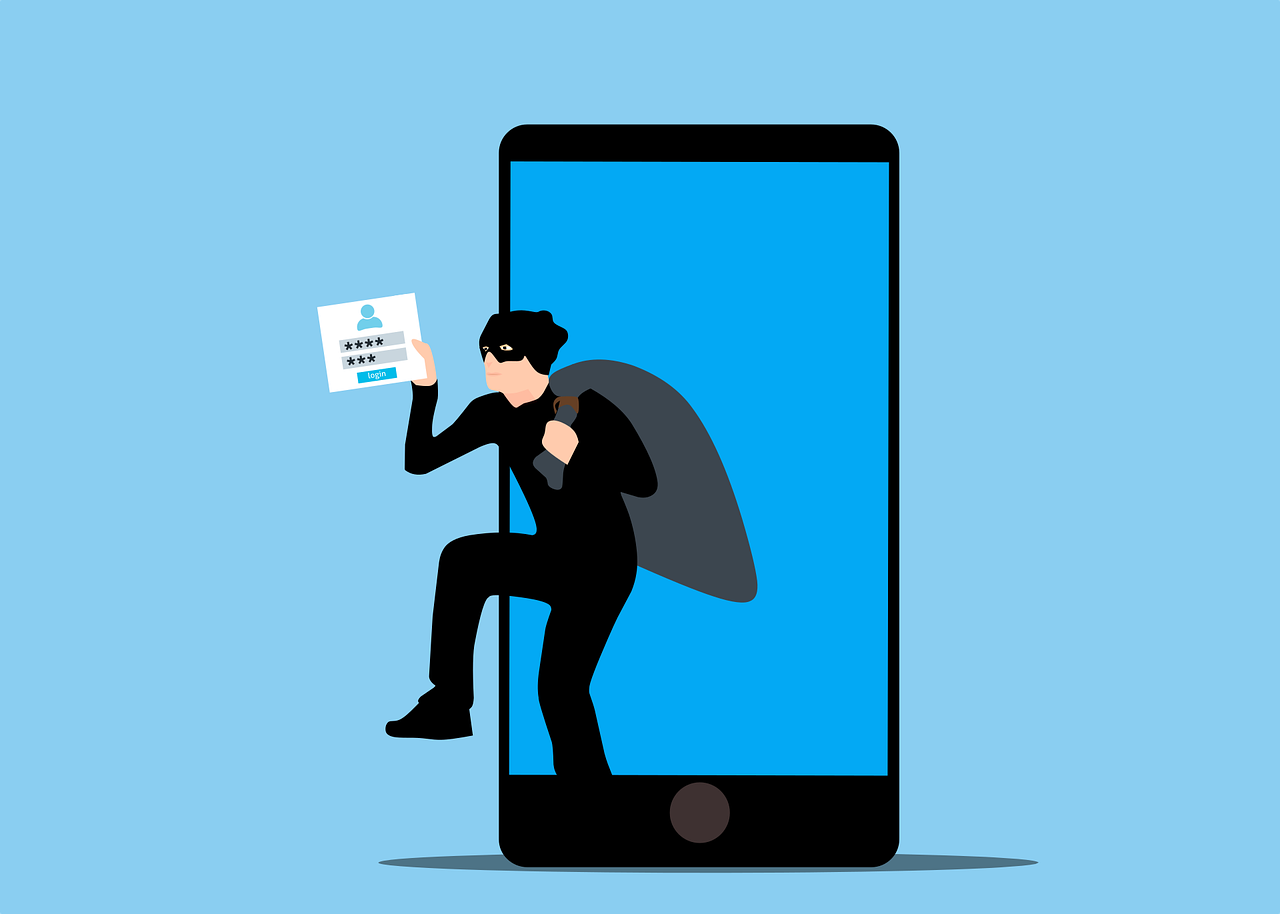
Understanding DMARC Records: Protecting Your Domain from Email Threats
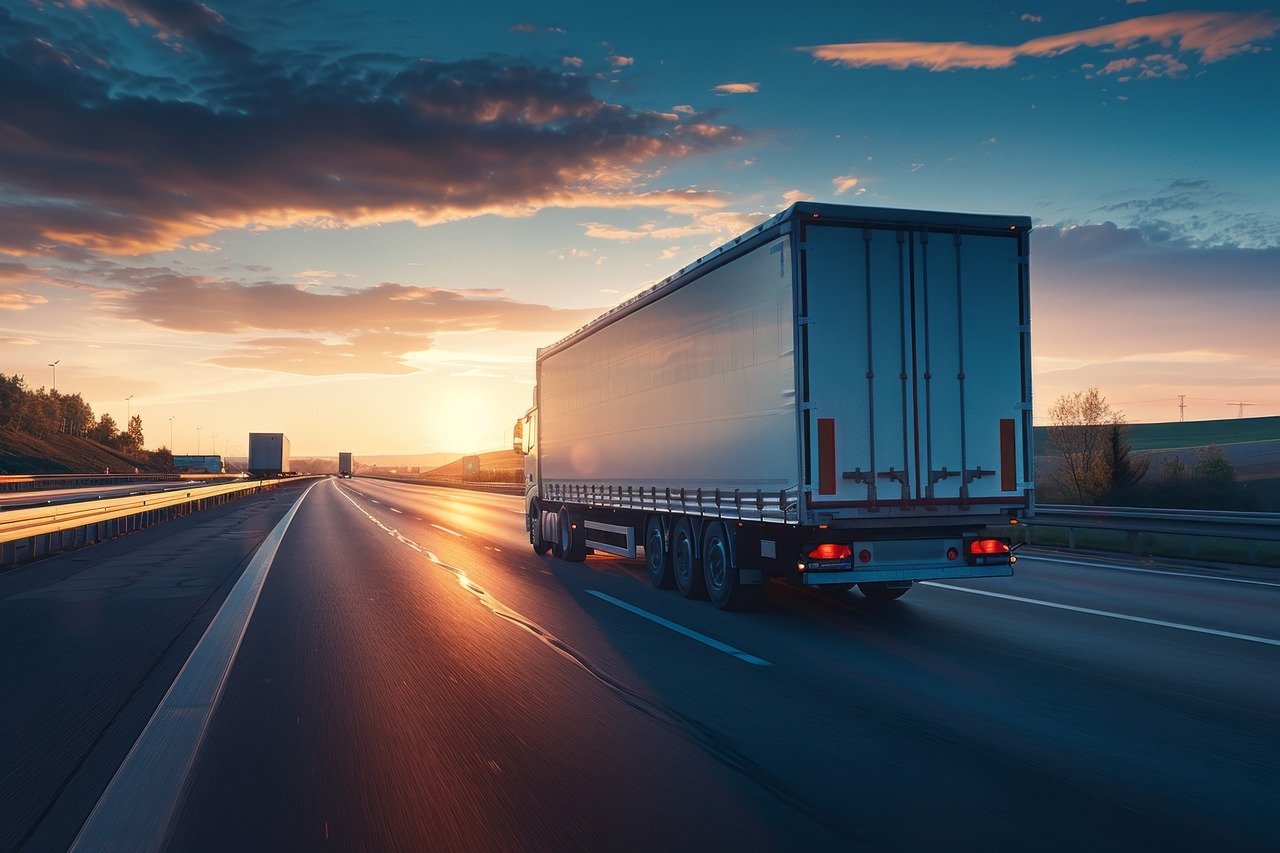
Transforming Fleet Management with AI and ML: A Guide to Optimization
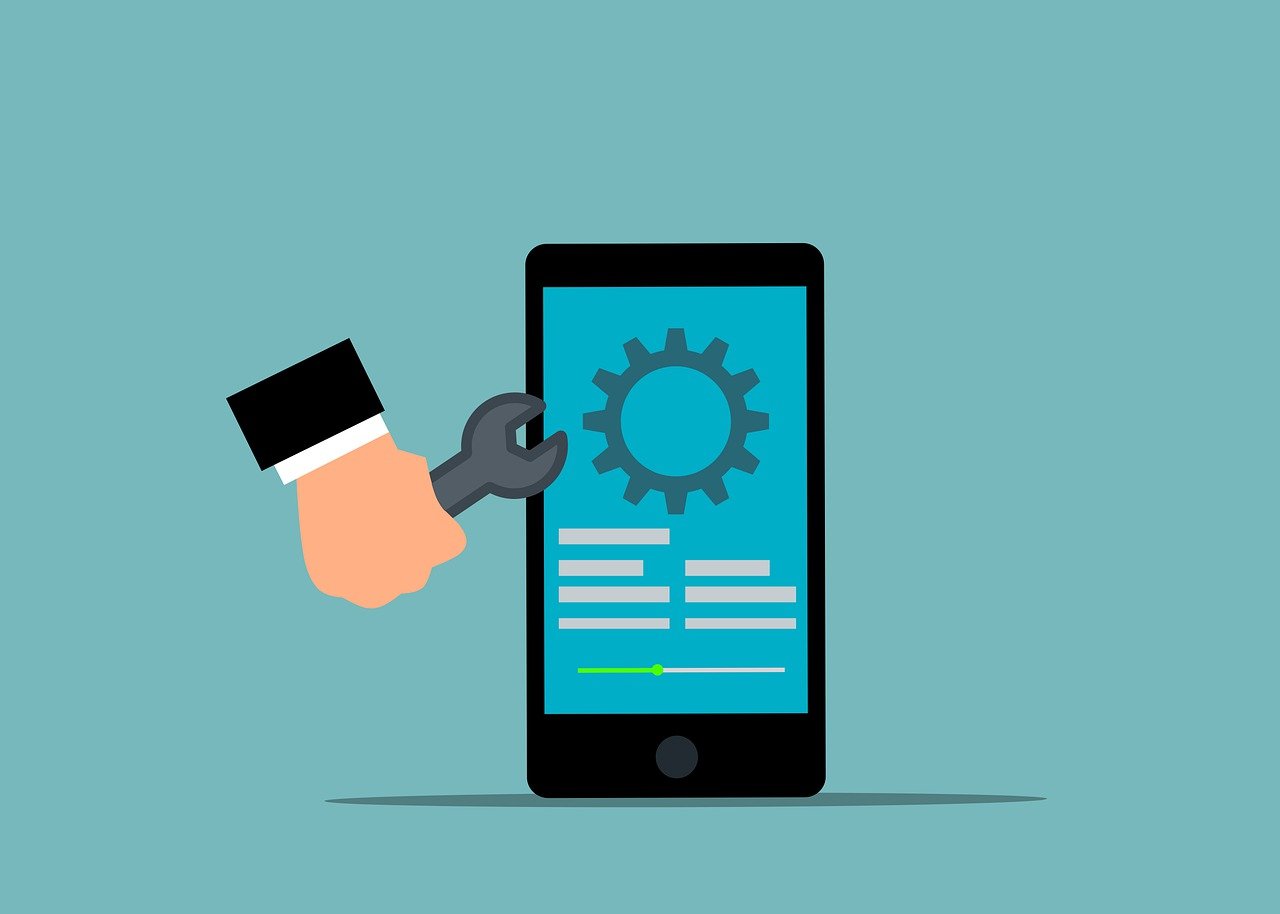
The Importance of Keeping Your Systems, Frameworks, and Databases Upgraded
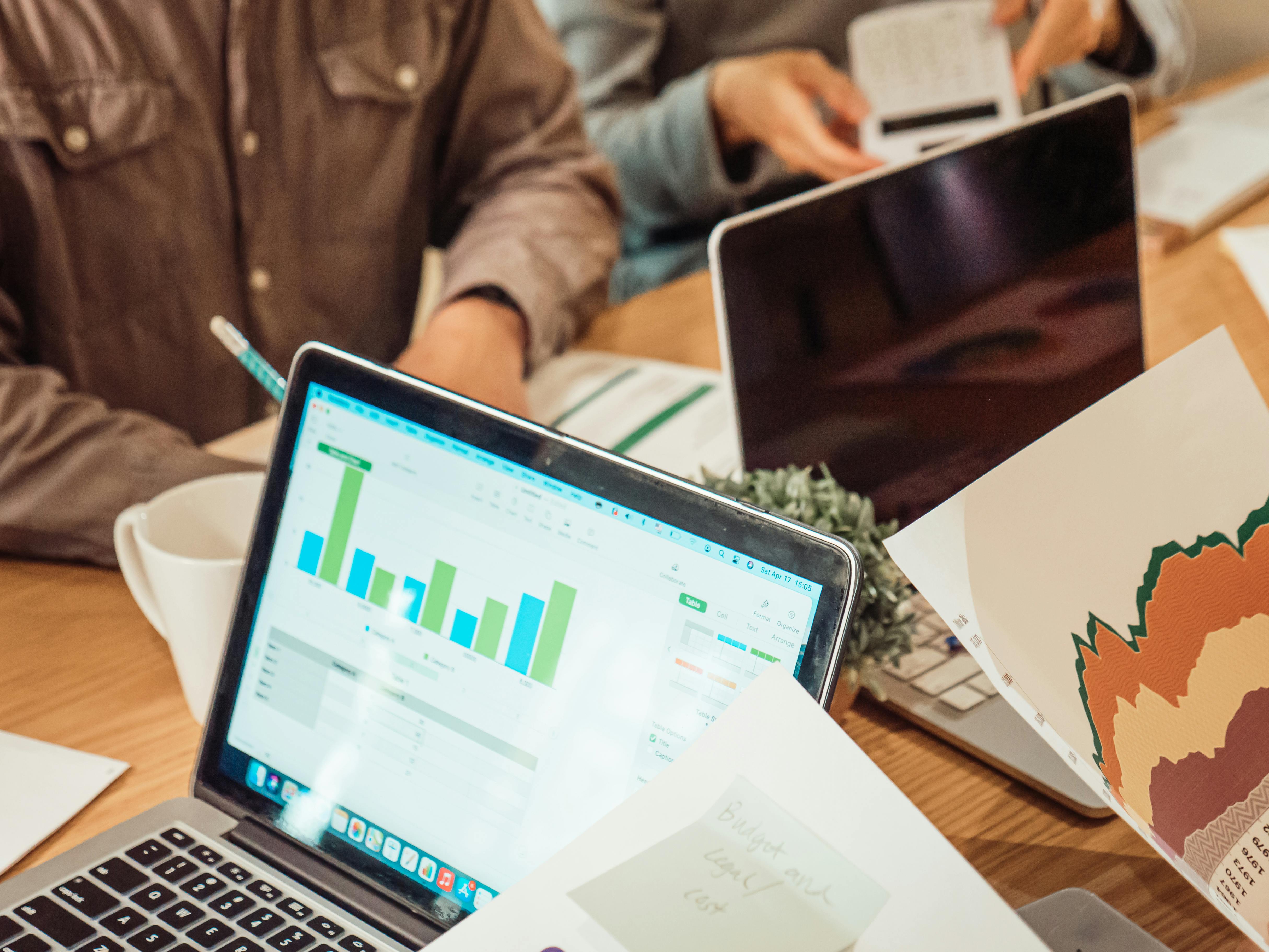
Leveraging Your Company’s Data to Make Smarter Decisions and Boost Productivity with AI and ML
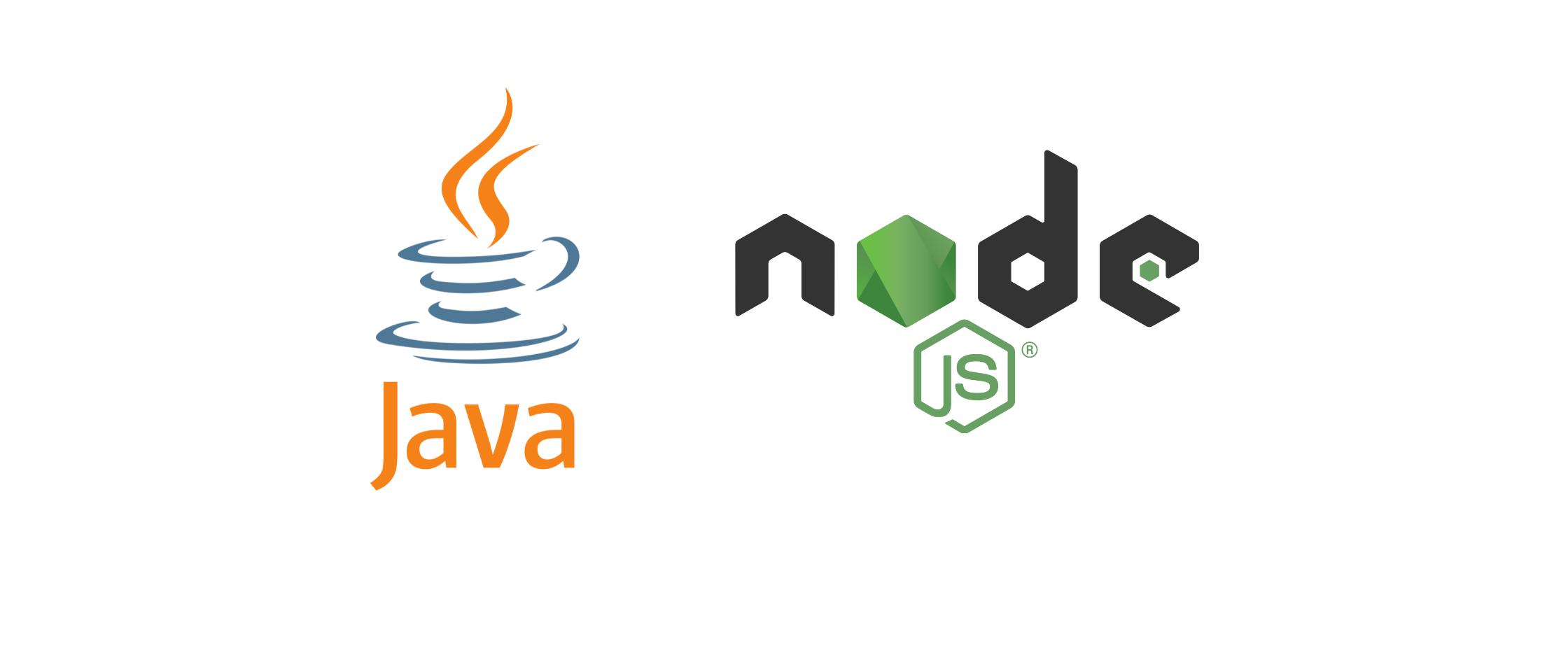