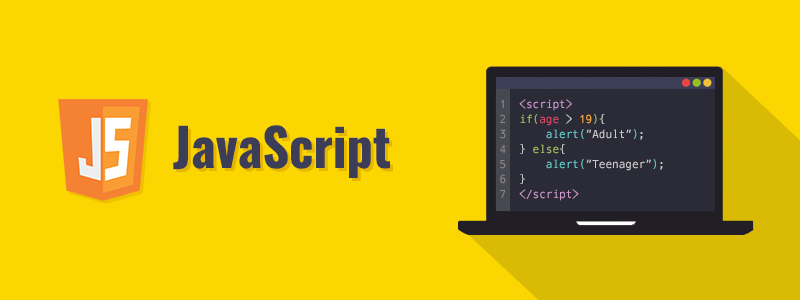
Javascript For Loops
Javascript For Loops in the ECMA Standard Simple For Loop The simplest type of for loop increments a variable as its iteration method. The variable
Object-Oriented Programming (OOP) is a programming paradigm that revolves around the concept of “objects.” Java, a widely-used programming language, is a great platform for learning and implementing OOP principles. In this article, we’ll dive into the fundamentals of OOP in Java, explaining key concepts with practical examples.
At the heart of OOP are the following fundamental concepts:
Objects are the building blocks of OOP. They represent real-world entities or concepts in your code. For example, you could have an Employee
object, a Car
object, or a BankAccount
object.
Classes serve as blueprints for creating objects. They define the structure and behavior that objects of a particular type will exhibit. For example, a Car
class defines what properties and methods an individual car object will have.
Encapsulation is the practice of bundling data (attributes) and methods (functions) that operate on that data into a single unit (i.e., a class). It’s about hiding the internal state of an object and allowing controlled access to it.
Inheritance is a mechanism that allows a new class (subclass or derived class) to inherit properties and behaviors from an existing class (superclass or base class). It promotes code reuse and the creation of a hierarchy of classes.
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It facilitates method overriding, where a subclass provides a specific implementation of a method defined in its superclass.
Let’s illustrate these concepts with some Java code examples:
public class Car {
// Attributes or fields
String make;
String model;
int year;
// Constructor
public Car(String make, String model, int year) {
this.make = make;
this.model = model;
this.year = year;
}
// Method
public void start() {
System.out.println("The car is starting.");
}
}
In this example, we’ve defined a Car
class with attributes (make
, model
, and year
), a constructor, and a start
method.
public class ElectricCar extends Car {
int batteryCapacity;
public ElectricCar(String make, String model, int year, int batteryCapacity) {
super(make, model, year); // Call the superclass constructor
this.batteryCapacity = batteryCapacity;
}
@Override
public void start() {
System.out.println("The electric car is starting silently.");
}
public void charge() {
System.out.println("Charging the electric car.");
}
}
Here, we’ve created a subclass ElectricCar
that inherits from the Car
superclass. We’ve also overridden the start
method and added a new method, charge
.
public class Main {
public static void main(String[] args) {
Car car1 = new Car("Toyota", "Camry", 2022);
ElectricCar car2 = new ElectricCar("Tesla", "Model 3", 2022, 75);
car1.start();
car2.start(); // Polymorphism in action
}
}
In this example, we create instances of both Car
and ElectricCar
and demonstrate polymorphism by invoking the start
method on both objects.
Object-Oriented Programming in Java provides a structured and efficient way to model and solve complex problems. It encourages the creation of reusable and maintainable code by organizing data and behavior into classes and objects. As you continue your journey in Java and OOP, remember to practice, explore more advanced concepts (like interfaces and abstract classes), and apply OOP principles to real-world projects to solidify your understanding.
Javascript For Loops in the ECMA Standard Simple For Loop The simplest type of for loop increments a variable as its iteration method. The variable
Retriving a List of attribute (field) values from a Object List (Array List) List users=new ArrayList<>(); List userIds=users .stream() .map(u->u.getId()) .collect(Collectors.toList()); Filter Objects by Attribute
let message = ‘helloWorld’ let convertedMessage=message.replace(/[A-Z]/g, letter => `_${letter.toLowerCase()}`); console.log(convertedMessage); output -> hello_world How to Convert Camel Case to Snake Case JavaScript
Things to know about the latest Kali release. 1. New Tools Here are all the new tools that come with the release. BruteShark – Network Analysis
In bubble sort ,an array is traversed from first element to last element. Then, current element is compared with the next element. If current element
Using yarn: yarn global add pm2 Using npm npm install pm2 -g Using debian apt update && apt install sudo curl && curl -sL