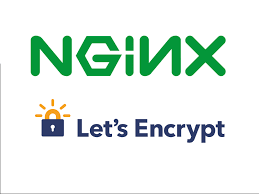
Lambda functions in Java, introduced in Java 8, are a way to define anonymous methods that can be passed around as arguments to other methods or stored in variables. This feature promotes functional programming, making the code more concise and readable.
A lambda function in Java has the following syntax:
(parameters) -> expression
Or, if the body contains multiple statements:
(parameters) -> {
// body
}
Here’s a simple example of a lambda function that takes two integers and returns their sum:
(int a, int b) -> a + b
In more complex scenarios, the lambda function can contain multiple statements:
(int a, int b) -> {
int sum = a + b;
return sum;
}
Example Implementations
Example 1: Basic Usage with Functional Interfaces
Java provides functional interfaces, which are interfaces with a single abstract method. One common functional interface is Runnable
.
Runnable runnable = () -> System.out.println("Hello, Lambda!");
runnable.run();
Example 2: Using Lambda with Collections
Lambda functions are often used with collections, especially with methods like forEach
, filter
, and map
.
- forEach
List names = Arrays.asList("John", "Jane", "Jack", "Doe");
names.forEach(name -> System.out.println(name));
- filter
List names = Arrays.asList("John", "Jane", "Jack", "Doe");
List filteredNames = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
filteredNames.forEach(System.out::println);
3. map
List names = Arrays.asList("John", "Jane", "Jack", "Doe");
List nameLengths = names.stream()
.map(String::length)
.collect(Collectors.toList());
nameLengths.forEach(System.out::println);
Real-Life Use Cases of Lambda Functions
1. Event Handling in GUI Applications
Lambda functions simplify event handling in graphical user interface (GUI) applications. For example, in JavaFX, lambda expressions make the code more readable and concise:
Button button = new Button("Click me");
button.setOnAction(event -> System.out.println("Button clicked!"));
2. Parallel Processing with Streams
Lambda functions are pivotal in parallel processing using the Stream API. They enable efficient data processing by simplifying the syntax and making the code more maintainable.
List numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List evenNumbers = numbers.parallelStream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
evenNumbers.forEach(System.out::println);
3. Sorting Collections
Lambda expressions simplify the process of sorting collections. Using Comparator
, you can define sorting criteria directly in the lambda function.
List names = Arrays.asList("John", "Jane", "Jack", "Doe");
names.sort((a, b) -> a.compareTo(b));
names.forEach(System.out::println);
Conclusion
Lambda functions have significantly changed the way Java developers write code, promoting a functional programming style. By enabling more concise, readable, and maintainable code, they have become an essential feature for modern Java development. Whether you are working with collections, handling events, or processing data in parallel, lambda functions offer a powerful and flexible tool to streamline your code.