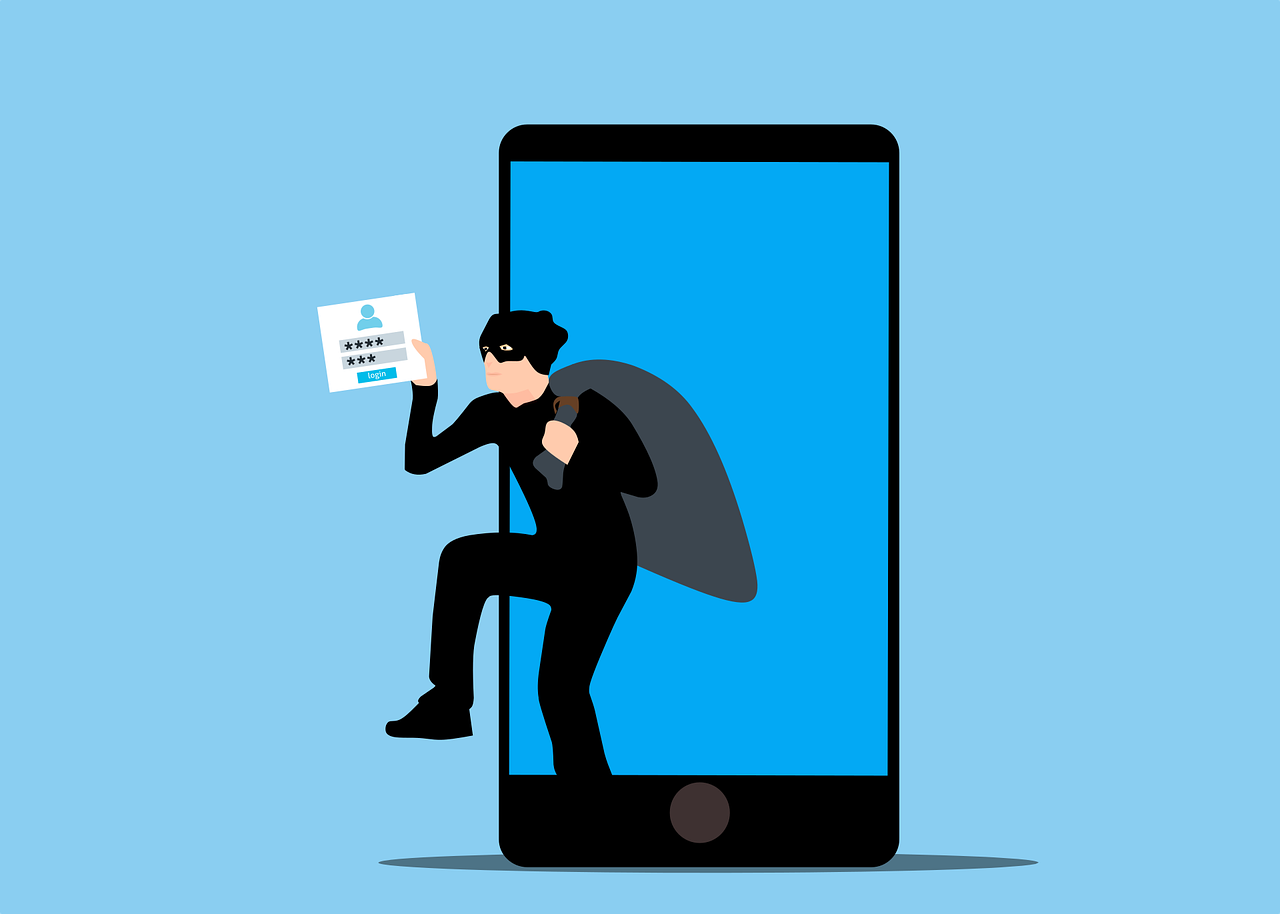
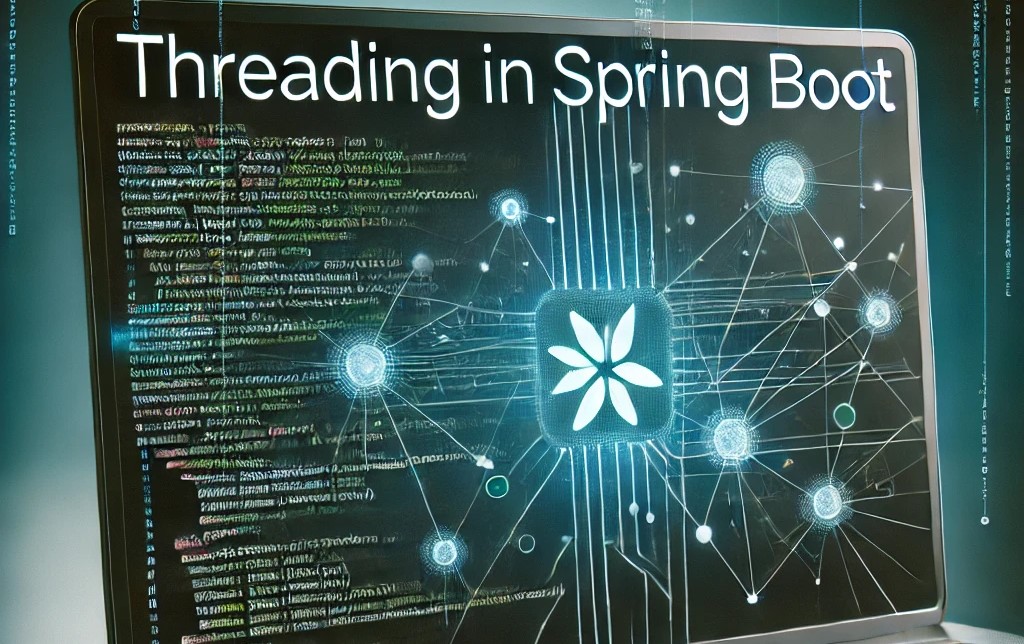
As software complexity grows, optimizing for speed and efficiency becomes essential. One tool to consider is threading—allowing tasks to run concurrently within a program. Here’s when and why to use threads effectively.
1. Handling Multiple I/O Operations
Threads shine in I/O-heavy applications where tasks wait for external resources (like file or network access). By using threads, other tasks can proceed without waiting for I/O operations to complete, improving response times.
2. Parallelizing Independent Tasks
If a program has independent tasks, threading enables these to run simultaneously. For example, web servers handling multiple client requests benefit from threading, as each request can be processed without waiting on others.
3. Background Tasks in User Interfaces
In applications with graphical interfaces, threading prevents the UI from freezing by running time-consuming processes in the background. This approach ensures a smooth user experience, as the main interface remains responsive while tasks like data loading occur in the background.
4. Data Processing and Computation-Heavy Applications
Although threads can help parallelize CPU-bound tasks, there are caveats. Threading is best suited for CPU-bound tasks if your program can avoid bottlenecks like memory access contention. For intensive calculations, consider parallel programming frameworks or languages specifically designed for high-performance computing.
5. Real-Time Applications
Real-time systems like games, robotics, and multimedia applications often rely on threading to handle simultaneous tasks (e.g., rendering graphics while processing user input). This concurrency is crucial in maintaining performance and meeting time-sensitive requirements.
Best Practices for Threading
- Limit Thread Count: Excessive threads can slow down the system; a balance must be struck to avoid overloading resources.
- Consider Thread Safety: Managing shared data across threads requires careful synchronization to prevent race conditions or deadlocks.
- Use Threading Libraries: Libraries such as Python’s
threading
module or Java’sExecutorService
simplify thread management and can reduce errors.
Threads can offer immense benefits when used appropriately, but they’re not a one-size-fits-all solution. By understanding when to apply them, developers can enhance application performance and maintain smoother user experiences.
Threading in Spring Boot
Spring Boot offers robust support for multithreading, primarily through its asynchronous features and task scheduling. You can enable asynchronous processing by annotating methods with @Async
, allowing them to run in the background without blocking the main application thread. Spring Boot uses thread pools to manage and control the number of concurrent threads, preventing resource overuse.
When to Use Threading in Spring Boot
Asynchronous Processing: For tasks like sending emails or processing files, where results aren’t immediately needed. Annotate methods with
@Async
to enable asynchronous execution.Scheduled Tasks: Use
@Scheduled
to run tasks at fixed intervals (e.g., clearing cache or sending periodic notifications). This is useful for jobs that should operate independently of other processes.Parallel Data Processing: In services that interact with multiple external APIs, you can use threading to make concurrent API calls, reducing wait times and improving user experience.
Best Practices
- Thread Pool Configuration: Customize thread pools to optimize performance based on your application’s needs. Configurations can be managed in
application.properties
for efficient resource usage. - Error Handling: Handle exceptions properly in asynchronous methods, as they won’t propagate back to the main thread by default.
- Thread Safety: Use
@Transactional
carefully, and avoid shared mutable state unless you’re using proper synchronization mechanisms.
Threading in Spring Boot can improve responsiveness and scalability, especially in applications that handle multiple simultaneous tasks or process background jobs. Proper configuration and error management will ensure your multithreaded Spring Boot application remains efficient and stable.
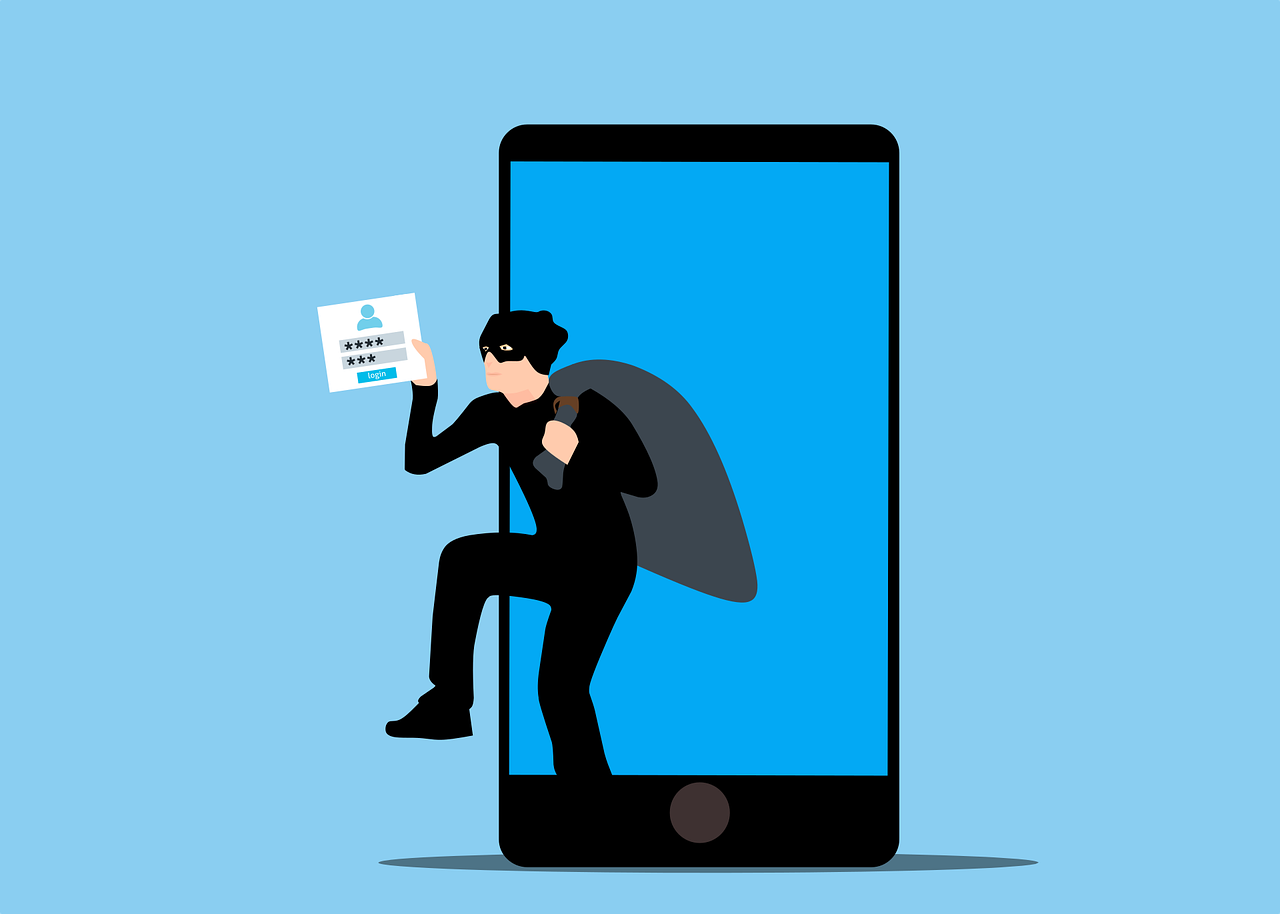
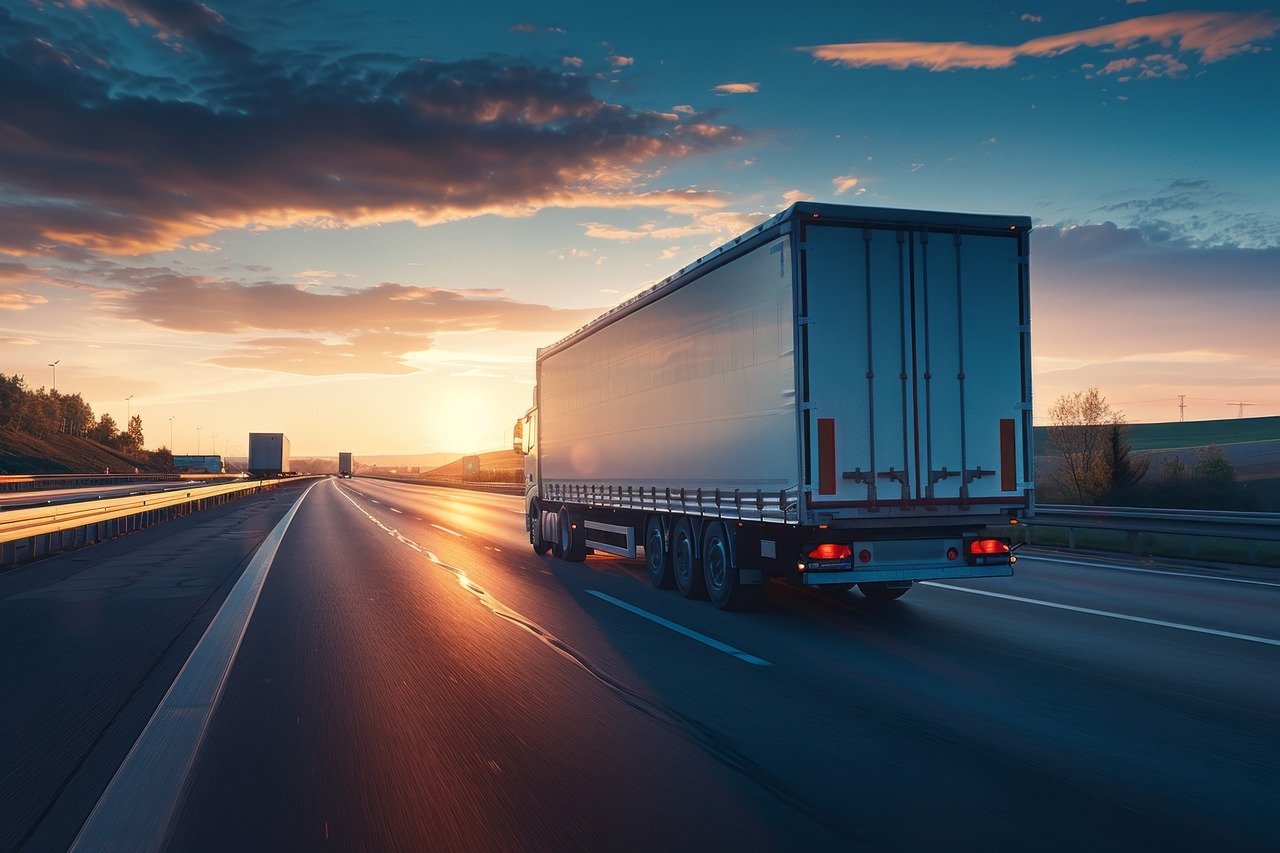
Transforming Fleet Management with AI and ML: A Guide to Optimization
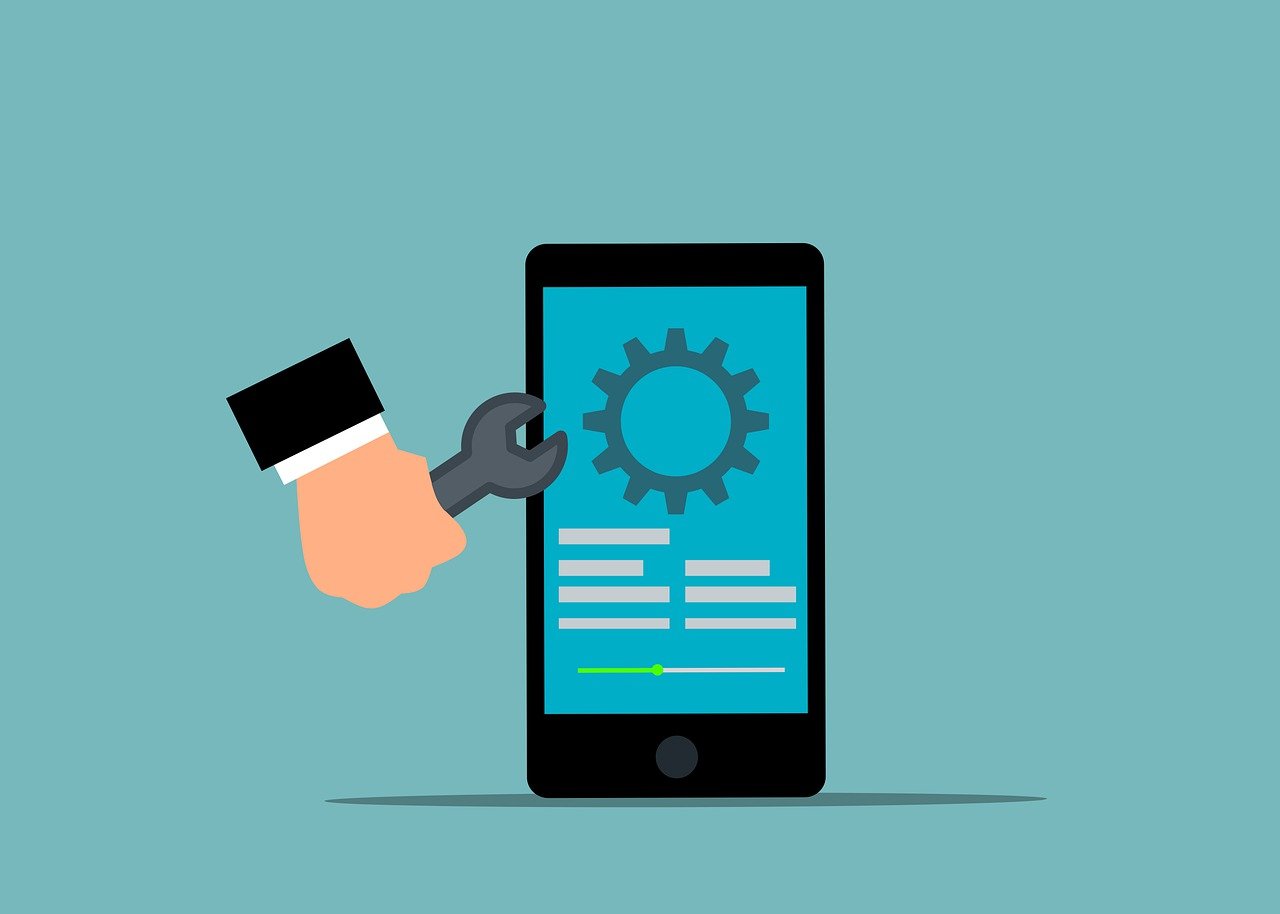
The Importance of Keeping Your Systems, Frameworks, and Databases Upgraded
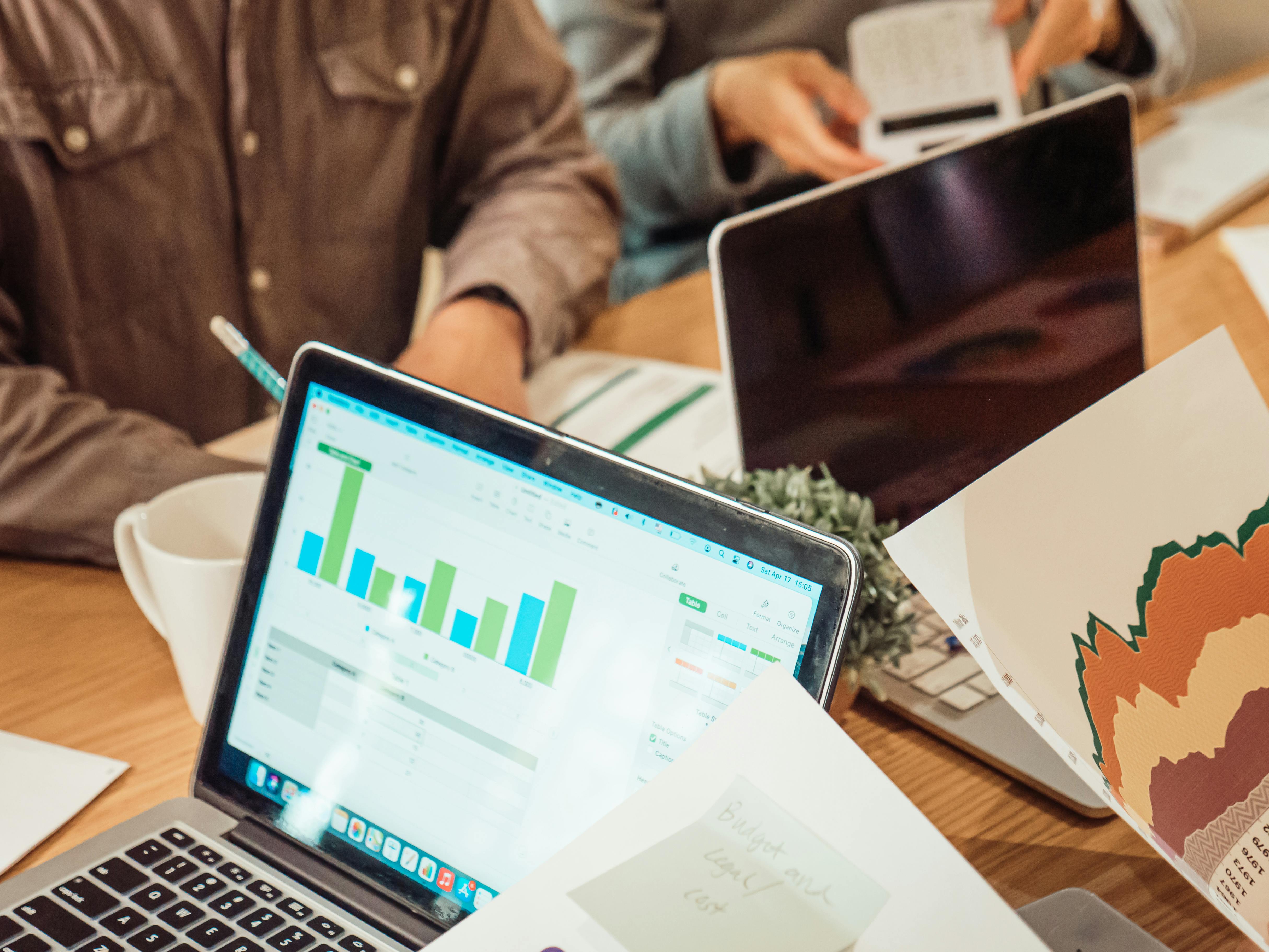
Leveraging Your Company’s Data to Make Smarter Decisions and Boost Productivity with AI and ML
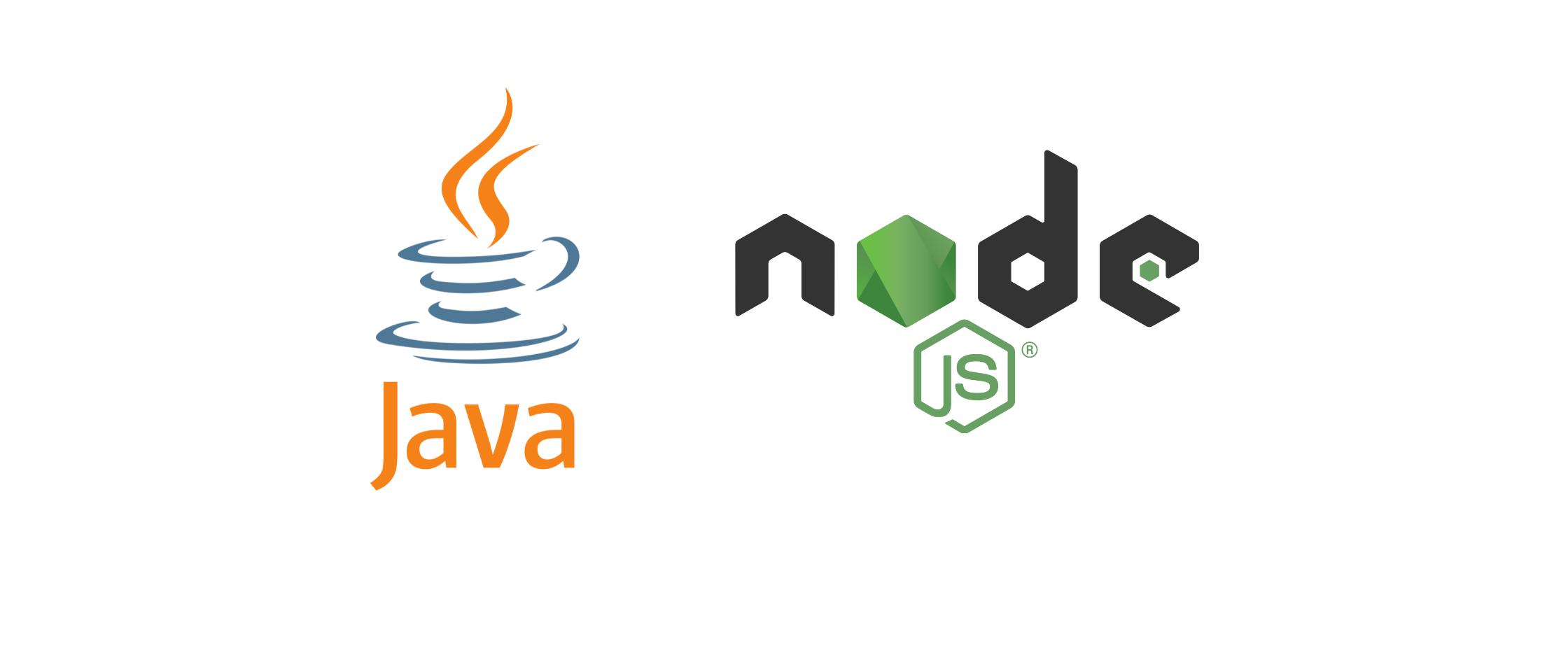
Choosing Between Node.js and Java for Your Backend Framework: A Strategic Perspective
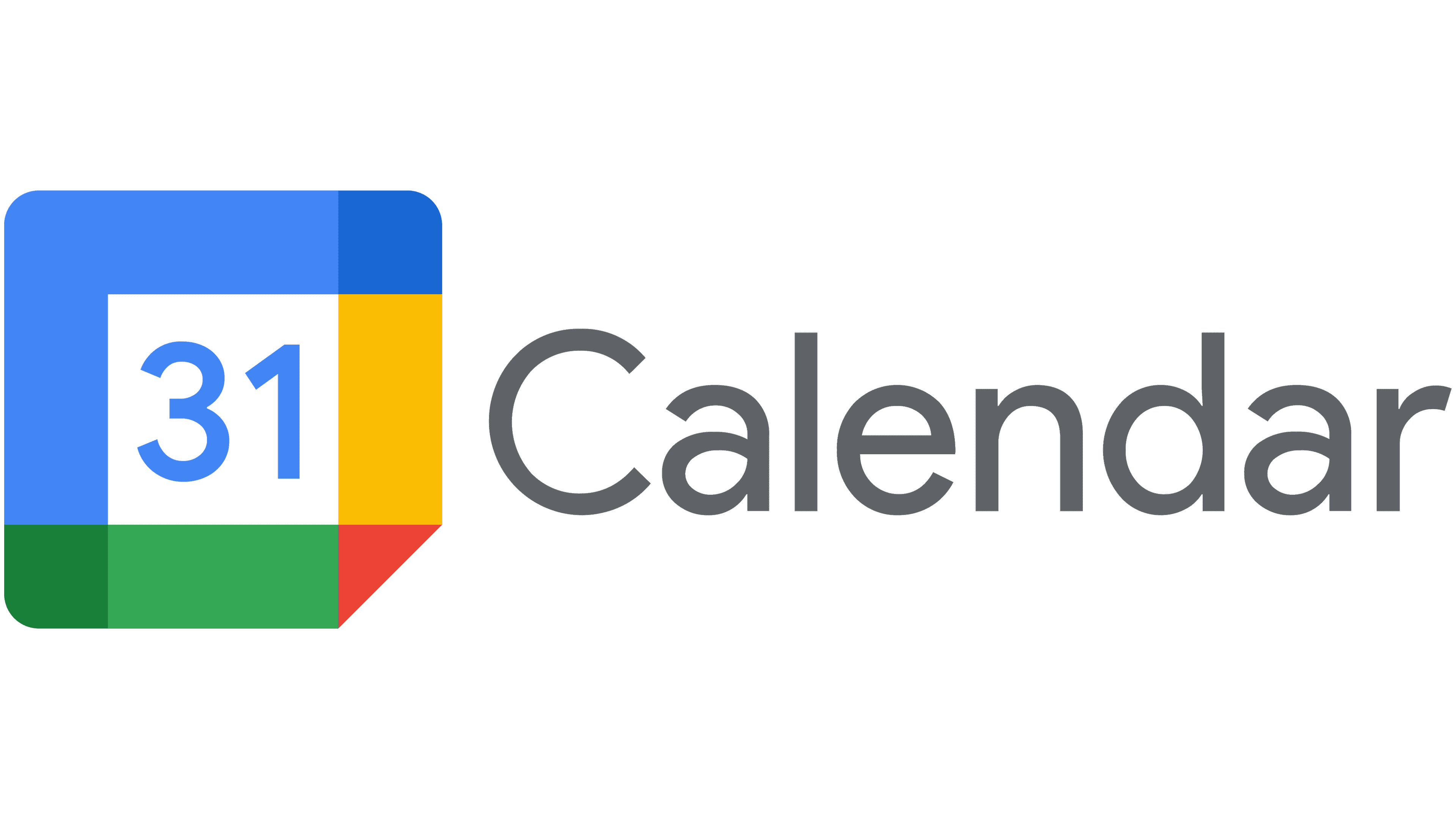